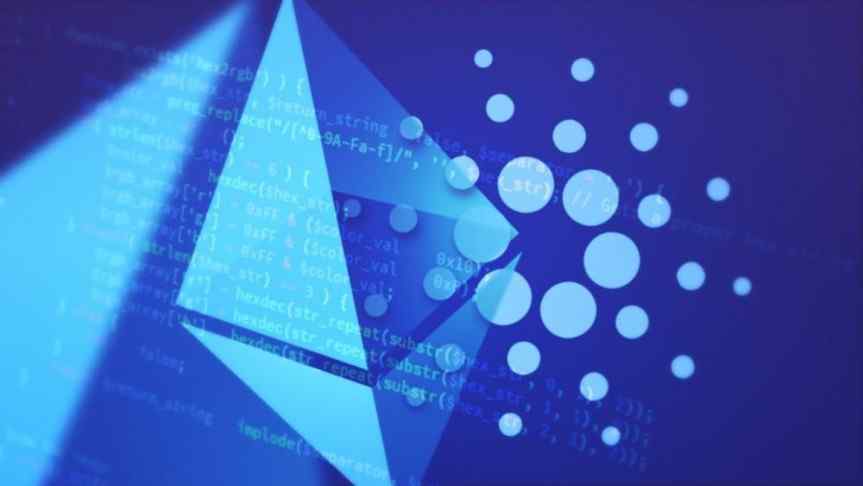
Smart Contracts and Means of Expressing Them: Ethereum's Vyper and Cardano's Plutus
May 8, 2018, 8:26AM
A brief summary of smart contract languages and their code-executing virtual environments including EVM, Solidity, Vyper, and Plutus.
Smart contracts are a natural evolution of distributed ledger technology. A smart contract is essentially a small program that executes predefined instructions to be triggered whenever certain conditions within the system are met (following an if-this-then-that conditional logic).
The concept was first articulated around 1996 by Nick Szabo, a software engineer and law scholar who was loosely associated with the cypherpunk movement at the time. Szabo broadly described smart contracts as a way of formalizing and securing relationships on public networks. Perhaps the most important property of smart contracts is that once deployed on a blockchain, they cannot be rolled back or changed. A smart contract is immutable and autonomous. In other words, it is a trustless system in which the fulfillment of an agreement between parties is automated.
Smart contracts are sometimes structured much like synthetic assets and financial instruments, such as derivatives or options. They can also be composed of self-enforceable legal agreements that bind the parties involved to fulfill obligations that they have previously committed to. Such contracts can thereby eliminate the burden of a lawsuit. (Projects like Open Law and iOlite are working towards formalizing and standardizing blockchain legalese).
Bundles of smart contracts can form more complex applications (DApps) and forge institutional bodies on a blockchain such as decentralized autonomous organizations (DAOs). This has set the stage for the smart contract to emerge as a programming discipline in its own right. In the following paragraphs, we'll briefly explore some of the trends developing in formalizing smart contract languages.
The Ethereum Virtual Machine (EVM)
Ethereum succeeded Bitcoin as the first platform to provide for smart contract functionality. Ethereum's developers equipped the basic distributed ledger with what they call a virtual machine, (with all the nodes acting together as a global singleton), which serves as the run-time environment for the execution of smart contract codes. The EVM is a 256-bit register stack that handles system state and logic and is the fundamental underlying consensus mechanism of Ethereum.
Smart contracts compile to EVM bytecode (that maps to a set of opcodes) which executes deterministically -- that is, it produces the same predictable output for a given input. (This, however, does not mean it will always behave as intended). Any kind of language that compiles to EVM bytecode can be customized to work with Ethereum, although Solidity is presently the one most widely used.
While the basic implementation of the EVM is specified in the yellow paper, there are ongoing efforts to create an executable specification (KEVM) which provides for runtime verification of code correctness and makes it easier to prototype changes to the EVM. KEVM is based on the K framework - a semantic framework for programming language builders. (In other words, it's a programming language for building programming languages.)
Cardano's IOHK (Input Output Hong Kong) is also working on developing its own custom virtual machine (called IELE) which is based on the LLVM compiler toolchain in addition to the low-level, Haskell-based Plutus Core, down to which smart contracts on Cardano compile.
The end goal of these continuous efforts is to provide for a secure and reliable bridge of interoperability between the ecosystems of similar blockchain platforms.
Solidity
The EVM can be programmed in any language equipped with a compiler that breaks it down to its bytecode. However, to date, Solidity has established itself as the widely adopted mainstream standard. Solidity is a high-level language similar to Javascript (but with types) which makes use of conventions from web development, networking, object-oriented programming and even assembly.
Solidity is meant to allow for a wide range of possible applications, but perhaps above all it was conceived as a language for the development of decentralized applications for the Web3. There have been a number of problems and fixes along the way due to Solidity's permissive nature on the one hand and the paramount importance of security in smart contracts on the other.
contract Pyramid {
function DynamicPyramid() { owner=msg.sender; }
function CollectAllFees() { owner.send(collectedFees); }
}
An example of a known smart contract exploit which seizes contract ownership and drains it
As we learn from the mistakes along the way of mapping out the uncharted territories of decentralized systems design and development, new patterns for writing smart contracts are distilled to better meet specific needs and fulfill different criteria.
Ethereum Vyper: Auditable Python-like Smart Contracts
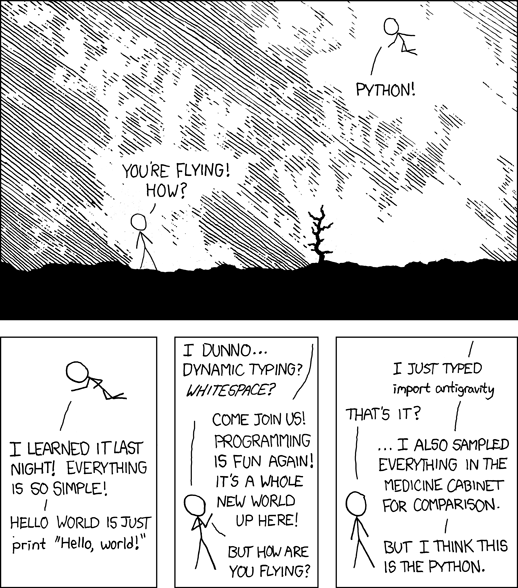
Vyper is a pythonic language for expressing smart contracts (using Python3 conventions) that targets the EVM and is the culmination of previous efforts in that direction (such as the deprecated Serpent preceding it). Unlike Solidity, Vyper is being developed as a minimalist and highly restrictive language with a focus on more trivial use cases (contractual agreements and scripts rather than complex DApps). This provides a more straightforward, pragmatic, and easily auditable regime for reading and writing smart contracts, which tries to clearly capture the intent of whoever writes the code.
Vyper's emphasis on readability and simplicity, along with the fact that Python as such is one of the best understood and most widely used programming languages, makes it most likely to be adopted as the de facto smart contracts language to be used by non-technical people.
Even more importantly, both Ethereum's sharding implementation and FFG -- its Proof-of-Stake iteration, are written in Vyper, which highlights the fact that Vyper is meant to be leveraged in the network’s next transition step towards maturation. The Ethereum Commonwealth (that maintains and develops Ethereum Classic) have also stated their intention to adopt Vyper as the default smart contracts language seeing as how it fits the Ethereum Classic ethos of "code is law".
@public
def transferFrom(_from: address, _to: address, _value: int128(uint256)) -> bool:
assert _value <= self.allowed[_from][msg.sender]
assert _value <= self.balances[_from]
self.balances[_from] -= _value # decrease balance of from address.
self.allowed[_from][msg.sender] -= _value # decrease allowance.
self.balances[_to] += _value # incease balance of to address.
return True
A function for transferring allowed tokens from one address to another in Vyper. The @public decorator exposes the function system-wide
In contrast to the permissiveness of Solidity, Vyper imposes strong typing and does away with inheritance (which makes audits difficult and messy), state modifiers, and things like recursive callings and operator overloading that open up gas limit attack vectors. Additionally, overflow checks for large number arithmetic operations are in place.
An online compiler and basic developer environment (with a set of example templates, including Casper) can be found here.
Cardano's Plutus: A Haskell-based Language With High-Security Assurances
Plutus is Cardano's Haskell derived language developed by IOHK. Plutus is a simplified, high-level adaptation of Haskell (in a way, what Vyper is to Python) that is strictly typed and uses formal specifications to ensure security and predictability. Haskell is perhaps the most popular of the purely functional programming languages. It is mostly known for its high degree of fault tolerance and has a reputation for being one of the most secure languages. It is commonly employed in mission-critical settings such as in aerospace companies and information systems of defense contractors, and it is the standard tool used to prototype cryptography by the NSA.
Given Cardano's goal to set the infrastructural highways for facilitating international trade and reducing operational costs and friction, developing a Haskell-driven platform only makes sense. Particularly in light of how financial institutions and electronic trading operations are increasingly gravitating towards functional programming which is best suited to their needs.
Of note, Haskell is closer to mathematics in style than it is to programming as commonly understood and practiced. Some of the defining features of a functional style programming are that there are no side effects (since they are explicit in the types), no states or mutations and, as a result, no implicit failures. This makes it easier to predict what a program is going to do upon execution. The formal verifications and specifications in Cardano's approach to design use pure logic to write down and define the description of what a program is supposed to do with maximal precision.
Plutus core is the underlying low-level code to which the user-facing Plutus proper is elaborated down to before compiling. This makes it possible for other Haskell-like languages to be built on top of Plutus core to accommodate specific needs and use cases as they arise. Cardano also uses something similar to Ethereum's gas, only in Cardano, more operations (opcodes) are free, although constrained as to how much one can use.
Some examples of Plutus code can be found here and a functional prototype of Plutus is accessible in IOHK's GitHub.
Conclusion
As the cryptocurrency space develops and matures, almost ten years after the launching of the Bitcoin blockchain, the efforts are increasingly channeled towards introducing common standards and cross-chain inter-operability. IOHK is at the forefront of coordinating those efforts (as well as housing projects like Ethereum Classic and ZenCash). Meanwhile, smart contract languages are entering the phase of becoming more formalized, simplified, and human-readable so as to make them more widely accessible to non-developers.
Disclaimer: information contained herein is provided without considering your personal circumstances, therefore should not be construed as financial advice, investment recommendation or an offer of, or solicitation for, any transactions in cryptocurrencies.